Next.js environment variables
In this article, we'll cover everything you need to know about environment variables and how to use them in Next.Js. We'll also discuss best practices for managing your environment variables and how to set them up on Vercel for your production deployments.
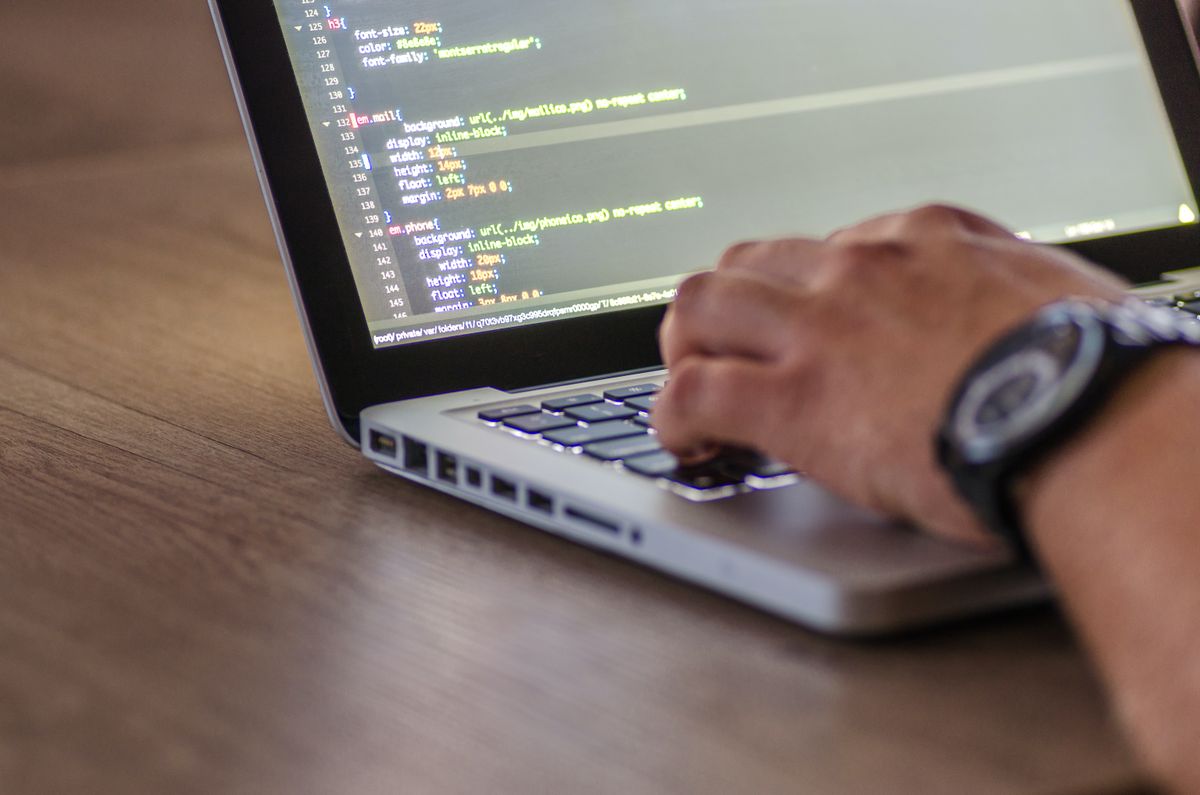
Introduction
As a Next.js developer, you need to have a good understanding of what environment variables are and how they work all the way from your local development to deploying to production. Environment variables are crucial for managing sensitive information such as API keys, database credentials, and other configuration settings. In this article, we'll cover everything you need to know about environment variables and how to use them in Next.Js. We'll also discuss best practices for managing your environment variables and how to set them up on Vercel for your production deployments.
What Are Environment Variables?
At the most basic form, environment variables are nothing more than Key/Value pairs. The magic sauce of environment variables is that they are used to store sensitive information such as database connection strings, or API keys. The reason that they can store sensitive information safely is because these values are never a part of your codebase; they live outside your code in a file named `.env`. Since they live outside of your code, they can be swapped out without having to manually change your code. For example,
MY_KEY=VALUE
Environment variable files should never be committed to Github or your remote repository. Make sure to add all environment variable files to your .gitignore file so that they are not tracked by git.
Setting Up Environment Variables in Next.js
To get started with environment variables in Next.js, you need to create a .env.local file in the root directory of your project. This file will contain all the environment variables that your application needs to function correctly for your local development environment. When deploying to production on Vercel, you will specify the production values on your project's dashboard on Vercel as we will see later. For example,
MY_API_KEY=123ABC!
Once you've added your environment variables to the .env file, you can access them in your Next.js backend code (getServerSideProps, getStaticProps, /api) using the process.env object. For example, to access the API key above, you can use the following code:
const apiKey = process.env.MY_API_KEY;
Client Side Environment Variables in Next.js
Environment variables that do not have the prefix `NEXT_PUBLIC_` (such as above) will not be able to be used in your client side code. This is by design. If you do try to access an environment variable on the client side of your Next.js code the environment variable will be `undefined`. In order to resolve the `undefined` issue you must add the `NEXT_PUBLIC_` prefix. This tells next.js that this variable is accessible from the client.
NOTE: Never set any secrets such as API keys or database connection strings to be client side environment variables. All client side variables are easily viewable on the frontend of your application.
Client side environment variables are usually used for configuration, such as which backend service to use.
NEXT_PUBLIC_API_URL: api.test.com
You could then retrieve this variable on the client
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
Best Practices for Managing Environment Variables in Next.js
A best practice for managing environment variables is to use different variables for different environments. For example, you may have different API keys for your development and production environments. By using different environment variables, you can ensure that your application is using the correct credentials for each environment. These variables can be stored in different `.env` files:
- `.env.local` - Local Development
- `.env.production` - Production
If you are deploying on Vercel you will only need to have one `.env.local` file. You will specify your production variables on your project's dashboard.
Production Environment Variables on Vercel
Setting up your application's envs on Vercel is a breeze. All you need to do is navigate to your projects settings and click `Environment Variables`. Here you are able to set up your key values just as you did for your `.env.local` file. Vercel also allows you to specify what environment you want to target for each variable. This is especially useful if you want your preview deployments to use a test database as opposed to your production database.
Conclusion
In conclusion, environment variables are an essential part of Next.js development & software development in general. They allow you to manage sensitive information and customize your application based on the environment. Now that you know how to use them, go build something great! 🚀